Shiro
Shiro能做什么?
Authentication 认证
Authorization 授权
Session Management 会话管理
Cryptgraphy 加密
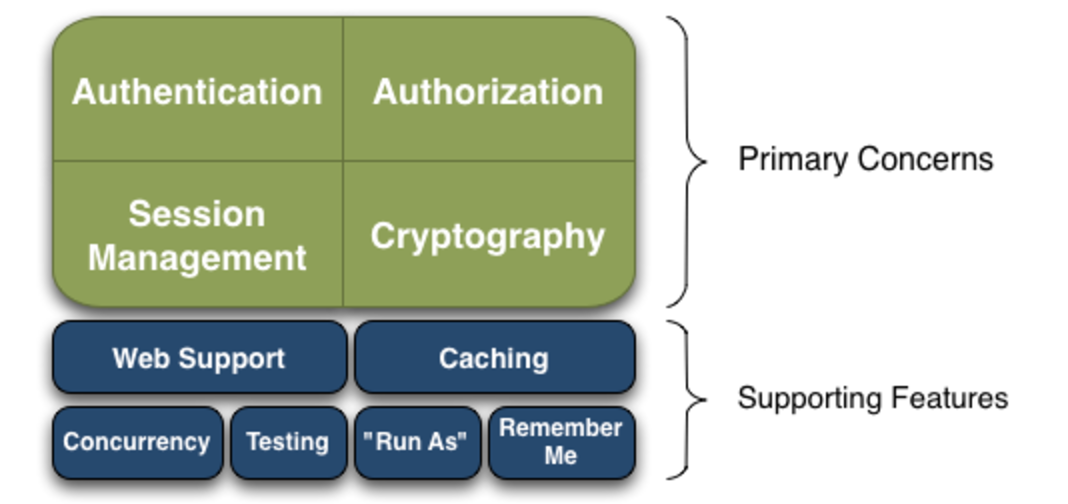
Shiro核心组件
Subject
当前用户(当前访问系统的第三方进程)
securityManager
内部组件管理
realm
封装了一套安全相关的DAO层的 “领域”,一组认证和授权
SpringBoot 集成
- Maven 依赖
1 2 3 4 5 6 7
| <dependency> <groupId>org.apache.shiro</groupId> <artifactId>shiro-spring</artifactId> <version>1.5.3</version> </dependency>
|
- 自定义ShiroConfig
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434
|
@Configuration public class ShiroConfig {
@Bean(name = "shirFilter") public ShiroFilterFactoryBean shiroFilterFactoryBean(@Qualifier("securityManager") SecurityManager securityManager) {
ShiroFilterFactoryBean shiroFilterFactoryBean = new ShiroFilterFactoryBean();
shiroFilterFactoryBean.setSecurityManager(securityManager); shiroFilterFactoryBean.setLoginUrl("/sessionInvalid"); shiroFilterFactoryBean.setSuccessUrl("/"); shiroFilterFactoryBean.setUnauthorizedUrl("/sessionInvalid");
LinkedHashMap<String, Filter> filtersMap = new LinkedHashMap<>(); filtersMap.put("kickout", kickoutSessionControlFilter()); shiroFilterFactoryBean.setFilters(filtersMap);
LinkedHashMap<String, String> filterChainDefinitionMap = new LinkedHashMap<>(); filterChainDefinitionMap.put("/logout", "logout"); filterChainDefinitionMap.put("/swagger-ui.html", "anon"); filterChainDefinitionMap.put("/webjars/**", "anon"); filterChainDefinitionMap.put("/swagger-resources/**", "anon"); filterChainDefinitionMap.put("/v2/**", "anon"); filterChainDefinitionMap.put("/u/**", "anon"); filterChainDefinitionMap.put("/swagger/**", "anon"); filterChainDefinitionMap.put("/doc.html", "anon"); filterChainDefinitionMap.put("/simpleBillRepertory/getShareStockUrl", "anon"); filterChainDefinitionMap.put("/alicheck", "anon"); filterChainDefinitionMap.put("/api-docs/**", "anon");
filterChainDefinitionMap.put("/**", "kickout,authc");
shiroFilterFactoryBean.setFilterChainDefinitionMap(filterChainDefinitionMap);
return shiroFilterFactoryBean; }
@Bean public ShiroRealm shiroRealm() { ShiroRealm shiroRealm = new ShiroRealm(); shiroRealm.setCachingEnabled(true); shiroRealm.setAuthenticationCachingEnabled(true); shiroRealm.setAuthenticationCacheName("authenticationCache"); shiroRealm.setAuthorizationCachingEnabled(true); shiroRealm.setAuthorizationCacheName("authorizationCache"); return shiroRealm; }
@Bean public CookieRememberMeManager cookieRememberMeManager() { CookieRememberMeManager cookieRememberMeManager = new CookieRememberMeManager(); cookieRememberMeManager.setCookie(simpleCookie()); cookieRememberMeManager.setCipherKey(Base64.decode("4AvVhmFLUs0KTA3Kprsdag==")); return cookieRememberMeManager; }
@Bean public EhCacheManager ehCacheManager() { EhCacheManager cacheManager = new EhCacheManager(); cacheManager.setCacheManagerConfigFile("classpath:ehcache-shiro.xml"); return cacheManager; }
@Bean("csRedisTemplate") public RedisTemplate<String, Serializable> csRedisTemplate(LettuceConnectionFactory redisConnectionFactory) { ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY); om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL); om.configure(MapperFeature.USE_GETTERS_AS_SETTERS, false); om.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
RedisTemplate<String, Serializable> template = new RedisTemplate<>(); template.setKeySerializer(new StringRedisSerializer()); template.setValueSerializer(new GenericJackson2JsonRedisSerializer(om)); template.setHashKeySerializer(new StringRedisSerializer()); template.setDefaultSerializer(new GenericJackson2JsonRedisSerializer(om)); template.setConnectionFactory(redisConnectionFactory); return template; }
@Bean("csRedisUtil") public CsRedisUtil csRedisUtil(@Qualifier("csRedisTemplate") RedisTemplate<String, Serializable> redisTemplate) { CsRedisUtil redisUtil = new CsRedisUtil(); redisUtil.setRedisTemplate(redisTemplate); return redisUtil; }
@Bean("shiroSessionManager") public ShiroSessionManager shiroSessionManager() {
ShiroSessionManager sessionManager = new ShiroSessionManager(); Collection<SessionListener> listeners = new ArrayList<>(); listeners.add(shiroSessionListener()); sessionManager.setSessionListeners(listeners); sessionManager.setSessionIdCookie(sessionIdCookie()); sessionManager.setSessionDAO(redisSessionDAO()); sessionManager.setCacheManager(ehCacheManager());
sessionManager.setGlobalSessionTimeout(60 * 1000 * 60); sessionManager.setDeleteInvalidSessions(true); sessionManager.setSessionValidationSchedulerEnabled(true); sessionManager.setSessionValidationInterval(60 * 1000 * 10); sessionManager.setSessionFactory(shiroSessionFactory());
sessionManager.setSessionIdUrlRewritingEnabled(false);
return sessionManager;
}
@Bean(name = "securityManager") public SecurityManager securityManager() { DefaultWebSecurityManager securityManager = new DefaultWebSecurityManager(); securityManager.setRealm(shiroRealm()); securityManager.setRememberMeManager(cookieRememberMeManager()); securityManager.setCacheManager(ehCacheManager()); securityManager.setSessionManager(shiroSessionManager()); return securityManager; }
@Bean public FormAuthenticationFilter formAuthenticationFilter() { FormAuthenticationFilter formAuthenticationFilter = new FormAuthenticationFilter(); formAuthenticationFilter.setRememberMeParam("rememberMe"); return formAuthenticationFilter; }
@Bean public KickoutSessionControlFilter kickoutSessionControlFilter() { KickoutSessionControlFilter kickoutSessionControlFilter = new KickoutSessionControlFilter(); kickoutSessionControlFilter.setCacheManager(ehCacheManager()); kickoutSessionControlFilter.setKickoutAfter(false); kickoutSessionControlFilter.setMaxSession(1); kickoutSessionControlFilter.setKickoutUrl("/login?kickout=1"); return kickoutSessionControlFilter; }
@Bean(name = "lifecycleBeanPostProcessor") public LifecycleBeanPostProcessor lifecycleBeanPostProcessor() { return new LifecycleBeanPostProcessor(); }
@Bean public RedisCacheManager redisCacheManager() { RedisCacheManager redisCacheManager = new RedisCacheManager(); redisCacheManager.setPrincipalIdFieldName("username"); redisCacheManager.setExpire(60 * 1000 * 60); return redisCacheManager; }
@Bean public RedisSessionDAO redisSessionDAO() { RedisSessionDAO redisSessionDAO = new RedisSessionDAO(); redisSessionDAO.setExpire(60 * 60); return redisSessionDAO; }
@Bean public SessionDAO sessionDAO() { RedisSessionDAO redisSessionDAO = redisSessionDAO(); redisSessionDAO.setCacheManager(ehCacheManager()); redisSessionDAO.setActiveSessionsCacheName("shiro-activeSessionCache"); redisSessionDAO.setSessionIdGenerator(sessionIdGenerator()); return redisSessionDAO; }
@Bean("sessionIdCookie") public SimpleCookie sessionIdCookie() { SimpleCookie simpleCookie = new SimpleCookie("sid"); simpleCookie.setHttpOnly(true); simpleCookie.setPath("/"); simpleCookie.setMaxAge(-1); return simpleCookie; }
@Bean public SessionIdGenerator sessionIdGenerator() { return new JavaUuidSessionIdGenerator(); }
@Bean("sessionFactory") public ShiroSessionFactory shiroSessionFactory() { ShiroSessionFactory sessionFactory = new ShiroSessionFactory(); return sessionFactory; }
@Bean("sessionListener") public ShiroSessionListener shiroSessionListener() { ShiroSessionListener sessionListener = new ShiroSessionListener(); return sessionListener; }
@Bean public SimpleCookie simpleCookie() { SimpleCookie simpleCookie = new SimpleCookie("rememberMe");
simpleCookie.setHttpOnly(true); simpleCookie.setPath("/"); simpleCookie.setMaxAge(2592000); return simpleCookie; }
@Bean public SimpleMappingExceptionResolver simpleMappingExceptionResolver() { SimpleMappingExceptionResolver simpleMappingExceptionResolver = new SimpleMappingExceptionResolver(); Properties properties = new Properties(); properties.setProperty("org.apache.shiro.authz.UnauthorizedException", "/unauthorized"); properties.setProperty("org.apache.shiro.authz.UnauthenticatedException", "/unauthorized"); simpleMappingExceptionResolver.setExceptionMappings(properties); return simpleMappingExceptionResolver; }
@Bean public MethodInvokingFactoryBean getMethodInvokingFactoryBean() { MethodInvokingFactoryBean factoryBean = new MethodInvokingFactoryBean(); factoryBean.setStaticMethod("org.apache.shiro.SecurityUtils.setSecurityManager"); factoryBean.setArguments(new Object[]{securityManager()}); return factoryBean; }
}
|
- 自定义realm
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class UserRealm extends AuthorizingRealm { @Override protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principalCollection) { SimpleAuthorizationInfo info = new SimpleAuthorizationInfo(); Subject subject = SecurityUtils.getSubject(); Object principal = subject.getPrincipal(); info.addStringPermission("user:add"); System.out.println("执行了授权"); return info; }
@Override protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken authenticationToken) throws AuthenticationException { System.out.println("执行了认证"); String username = "123"; String password = "123"; UsernamePasswordToken token = (UsernamePasswordToken)authenticationToken; if (!token.getUsername().equals(username)) { return null; } return new SimpleAuthenticationInfo("",password,null,"");
} }
|
- 自定义sessionManager
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| public class ShiroSessionManager extends DefaultWebSessionManager { private static final String AUTHORIZATION = "authToken"; private static final String REFERENCED_SESSION_ID_SOURCE = "Stateless request"; public ShiroSessionManager(){ super(); } @Override protected Serializable getSessionId(ServletRequest request, ServletResponse response){ String id = WebUtils.toHttp(request).getHeader(AUTHORIZATION); System.out.println("id:"+id); if(StringUtils.isEmpty(id)){ //如果没有携带id参数则按照父类的方式在cookie进行获取 System.out.println("super:"+super.getSessionId(request, response)); return super.getSessionId(request, response); }else{ //如果请求头中有 authToken 则其值为sessionId request.setAttribute(ShiroHttpServletRequest.REFERENCED_SESSION_ID_SOURCE,REFERENCED_SESSION_ID_SOURCE); request.setAttribute(ShiroHttpServletRequest.REFERENCED_SESSION_ID,id); request.setAttribute(ShiroHttpServletRequest.REFERENCED_SESSION_ID_IS_VALID,Boolean.TRUE); return id; } } }
|
需要在ShiroConfig中将自定义的SessionManager注入管理器中
1 2 3 4 5 6 7
| @Bean public DefaultWebSecurityManager defaultWebSecurityManager() { DefaultWebSecurityManager securityManager = new DefaultWebSecurityManager(); securityManager.setRealm(realm()); securityManager.setSessionManager(sessionManager()); return securityManager; }
|
- 自定义全局异常处理
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| public class GlobalExceptionResolver implements HandlerExceptionResolver { private static Logger logger = LoggerFactory.getLogger(GlobalExceptionResolver.class); @Override public ModelAndView resolveException(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) { ModelAndView mv; if(ex instanceof UnauthorizedException){ mv = new ModelAndView("/user/unauth"); return mv; }else { mv = new ModelAndView(); FastJsonJsonView view = new FastJsonJsonView(); BaseResponse baseResponse = new BaseResponse(); baseResponse.setMsg("服务器异常"); ex.printStackTrace(); logger.error(ExceptionUtils.getFullStackTrace(ex)); Map<String,Object> map = new HashMap<>(); String beanString = JSON.toJSONString(baseResponse); map = JSON.parseObject(beanString,Map.class); view.setAttributesMap(map); mv.setView(view); return mv; } } }
|
将自定义全局异常处理注入Shiro中
1 2 3 4 5 6 7 8
|
@Bean(name = "exceptionHandler") public HandlerExceptionResolver handlerExceptionResolver(){ return new GlobalExceptionResolver(); }
|